一、设置 http 请求语法
resp = requests.请求方法(url='URL地址', params={k:v}, headers={k:v}, data={k:v}, json={k:v}, cookies='cookie数据'(如:令牌)) 请求方法: get请求 - get() post请求 - post() put请求 - put() delete请求 - delete() url: 待请求的url - string类型 params:查询参数 - 字典 headers:请求头 - 字典 data:表单格式的 请求体 - 字典 json:json格式的 请求体 - 字典 cookies:cookie数据 - string类型 resp:响应结果
二、cookie
1、cookie:工程师 针对 http协议是无连接、无状态特性,设计的 一种技术。 可以在浏览器端 存储用户的信息。
2、特性:
cookie 用于存储 用户临时的不敏感信息。
cookie 位于浏览器(客户端)端。默认大小 4k(可以调整)
cookie 中的数据,可以随意被访问,没有安全性可言。
cookie 中存储的数据类型, 受浏览器限制。
3、Cookie+Session认证方式
在计算机中,认证用户身份的方式有多种!课程中接触 2种:
ihrm项目:token认证。
tpshop项目:cookie+Session认证。
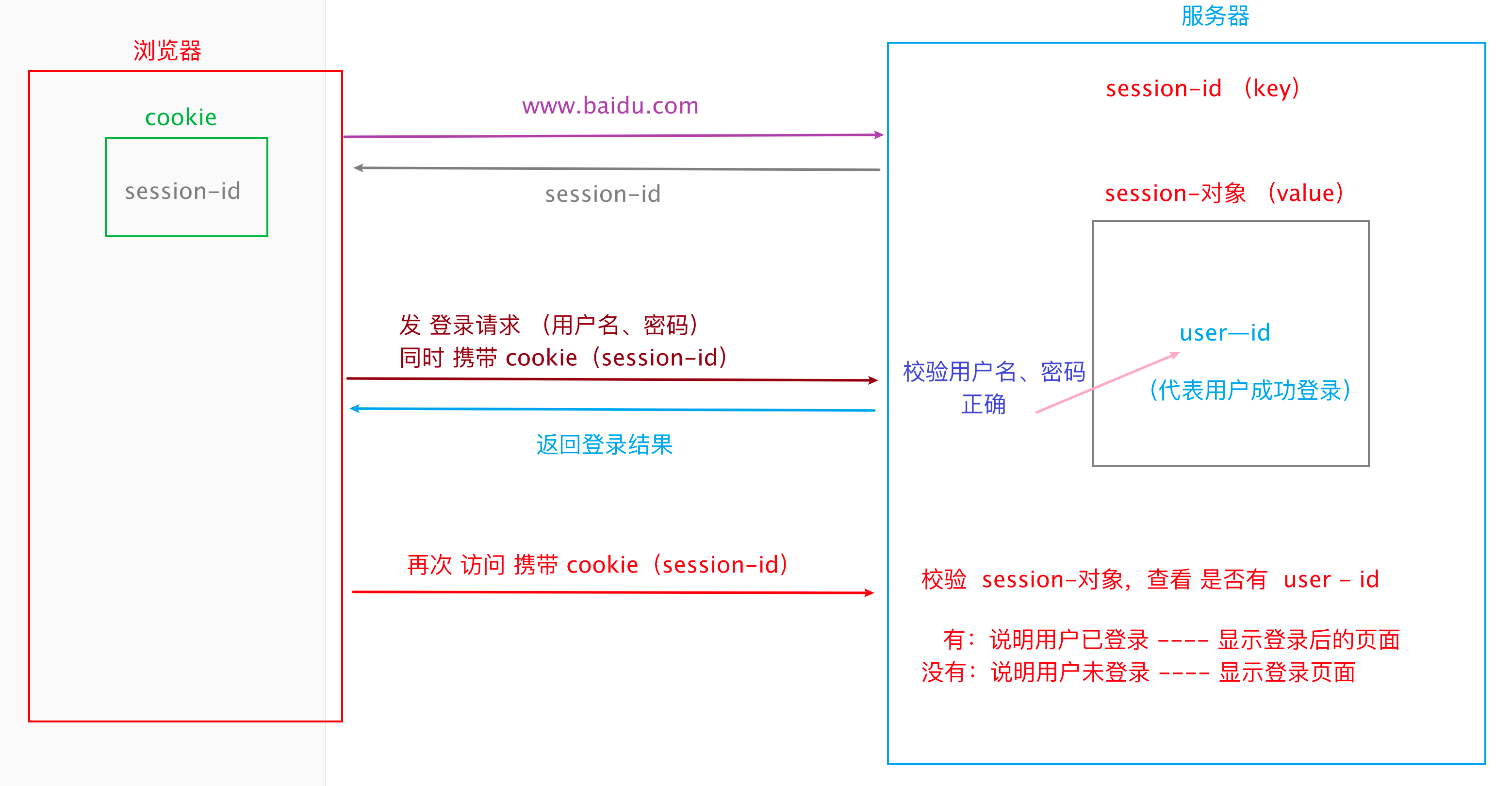
4、案例
完整实现 TPshop商城登录,并获取 “我的订单” 页面数据。
获取验证码:http://tpshop-test.itheima.net/index.php?m=Home&c=User&a=verify
登录:http://tpshop-test.itheima.net/index.php?m=Home&c=User&a=do_login
我的订单:http://tpshop-test.itheima.net/Home/Order/order_list.html
import requests # 发送 获取验证码请求 resp_v = requests.get(url="http://tpshop-test.itheima.net/index.php? m=Home&c=User&a=verify&r=0.21519623710645064") # 从 获取验证码 的响应结果,提取 cookie my_cookie = resp_v.cookies # 发送 post 登录请求 url、请求头、请求体。 携带 cookie。 得响应结果 resp = requests.post(url="http://tpshop-test.itheima.net/index.php? m=Home&c=User&a=do_login&t=0.7094195931397276", headers={"Content-Type": "application/x-www-form-urlencoded"}, data={"username": "13012345678", "password": "12345678", "verify_code": "8888"}, cookies=my_cookie) # 打印响应结果 print(resp.json()) # 发送 查看我的订单 请求 resp_o = requests.get(url="http://tpshop-test.itheima.net/Home/Order/order_list.html", cookies=my_cookie) print(resp_o.text)
三、session
1、介绍:也叫 会话。通常出现在网络通信中,从客户端借助访问终端登录上服务器,直到 退出登录 所产生的通信数据,保存在 会话中。
2、特性:
Session 用于存储 用户的信息。
Session 位于服务端。大小直接使用服务器存储空间
Session 中的数据,不能随意被访问,安全性较高。
Session 中存储的数据类型,受服务器影响,几乎能支持所有的数据类型。
3、Session自动管理Cookie
因为 Cookie 中的 数据,都是 Session 传递的。因此,Session 可以直接 自动管理 cookie
4、案例:
借助session重新实现 上述 TPshop商城登录,并获取 “我的订单” 页面数据。
1. 创建一个 Session 实例。
2. 使用 Session 实例,调 get方法,发送 获取验证码请求。(不需要获取cookie)
3. 使用 同一个 Session 实例,调用 post方法,发送 登录请求。(不需要携带 cookie)
4. 使用 同一个 Session 实例,调用 get方法,发送 查看我的订单请求。(不需要携带 cookie)
import requests # 1. 创建一个 Session 实例。 session = requests.Session() # 2. 使用 Session 实例,调 get方法,发送 获取验证码请求。(不需要获取cookie) resp_v = session.get(url="http://tpshop-test.itheima.net/index.php? m=Home&c=User&a=verify&r=0.21519623710645064") # 3. 使用 同一个 Session 实例,调用 post方法,发送 登录请求。(不需要携带 cookie) resp = session.post(url="http://tpshop-test.itheima.net/index.php? m=Home&c=User&a=do_login&t=0.7094195931397276", data={"username": "13012345678", "password": "12345678", "verify_code": "8888"}) print(resp.json()) # 4. 使用 同一个 Session 实例,调用 get 方法,发送 查看我的订单请求。(不需要携带 cookie) resp_o = session.get(url="http://tpshop-test.itheima.net/Home/Order/order_list.html") print(resp_o.text)